预定义全局数据
在设计器中,预定义全局数据可以为表单设计提供统一的数据源,便于全局管理和复用。通过导入预定义的全局数据,您可以在设计表单时复用相同的数据源,从而简化配置并提高开发效率。
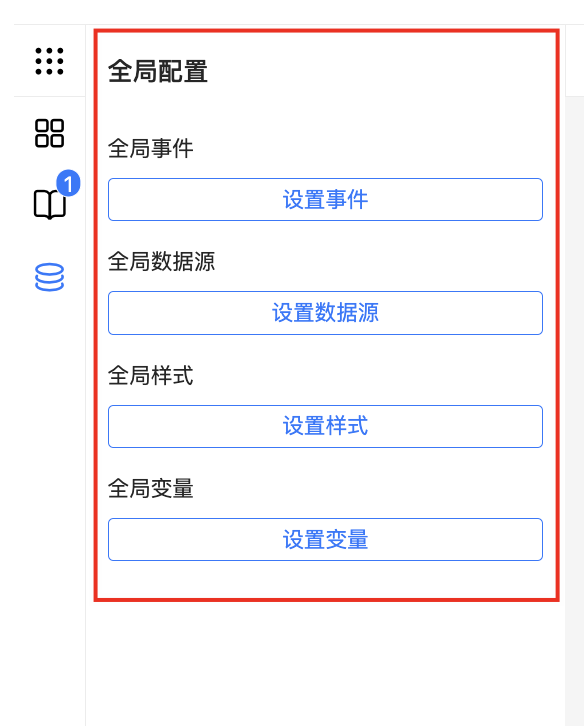
设置全局数据
在设计器中,可以通过对应的方式来导入预定义的全局数据。
vue
<template>
<fc-designer ref="designer" />
</template>
<script setup>
import { ref, onMounted } from 'vue';
const designer = ref();
onMounted(() => {
//导入全局事件
designer.value.setGlobalEvent({
"event_Feq4lui56zxbabc": {
"label": "自定义",
"deletable": false,
"handle": function ($inject) {
console.log($inject);
}
},
});
//导入全局变量
designer.value.setGlobalVariable({
"var_Fppdlz6gytmzb1c": {
"label": "token",
"deletable": false,
"handle": function (get, api) {
return get('$cookie.token') || 'default Token'
}
}
})
//导入全局样式
designer.value.setGlobalClass({
"cls_Fzmulzw3u0oib0c": {
"label": "fff",
"deletable": false,
"style": {
"color": "red"
}
}
})
//导入全局数据源
designer.value.setGlobalData({
"data_Fk6dlui4k0xuabc": {
"label": "自定义数据",
"type": "static",
"data": [
1,
2,
3,
4
]
},
"data_Fs1elui4kttlacc": {
"action": "http://192.168.1.4:8081/",
"deletable": false,
"method": "GET",
"headers": {},
"data": {},
"parse": function (res) {
return res.data;
},
"label": "自定义接口数据",
"type": "fetch"
}
})
});
</script>
设置外部变量描述
通过varList
配置项定义外部变量的描述,例如cookie
中的数据。方便用户理解和使用。
vue
<template>
<fc-designer ref="designer" :config="config" />
</template>
<script setup>
import { ref, onMounted } from 'vue';
const config = ref({
varList: [
{
id: 'category',
label: '分类'
},
{
id: '$cookie',
children: [
{
id: 'token',
label: '用户令牌'
},
{
id: 'user',
label: '用户信息',
children: [
{
id: 'name',
label: '用户名称'
}
]
},
]
}
]
});
</script>
数据结构
全局数据源
全局数据源用于提供表单中可能需要的各种数据。数据源可以是静态数据(如预定义的数据列表)或远程数据(通过远程 API 获取的数据)。
ts
//静态数据
interface StaticDataItem {
//数据名称
label: string;
//数据类型
type: 'static';
//数据
result: any;
}
//远程数据
interface FetchDataItem {
//数据名称
label: string;
//数据类型
type: 'fetch'
//请求链接
action: string,
//请求方式
method: 'GET' | 'POST',
//请求头部
headers?: Object,
//附带数据
data?: Object,
//远程数据解析
parse?: string | ((res: any) => any),
//远程异常处理
onError?: string | ((e) => void),
}
//全局数据源
export interface GlobalData {
[id:string] : StaticDataItem | FetchDataItem;
}
全局事件
全局事件用于定义可以在表单中触发的事件及其处理方式。
ts
interface GlobalEvent {
[id: string]: {
//数据名称
label: string;
//是否可以删除
deletable: boolean;
//回调事件
handle: string | (($inject: Object) => void);
}
}
全局样式
全局样式用于定义可以在表单中应用的样式类。
ts
interface GlobalClass {
[className: string]: {
//数据名称
label: string;
//是否可以删除
deletable: boolean;
//回调事件
style: Object;
}
}
全局变量
全局变量用于定义表单中需要使用的变量及其计算逻辑。
ts
export interface GlobalVariable {
[id: string]: {
//数据名称
label: string;
//是否可以删除
deletable: boolean;
//回调事件
handle: string | ((get: Function, api: Api) => any);
}
}
外部变量描述
外部变量描述用于定义通过setData
方法往表单中导入的外部数据
ts
type VarItem = {
id?: string;
label: string;
children?: VarItem[];
}
type VarList = VarItem[];
通过下列id
可以增加变量列表的默认选择项
- $cookie: 获取cookie中的数据
- $localStorage: 获取localStorage中的数据